Have you ever felt like your Vue.js applications are becoming increasingly complex and challenging to maintain? As your projects grow, the need for well-defined structures and predictable behaviors becomes paramount. This is where design patterns and best practices come into play, providing a blueprint for building robust, scalable, and maintainable Vue.js applications.
Image: github.com
This comprehensive guide will delve into the world of Vue.js 3 design patterns and best practices. We’ll explore common patterns like Model-View-ViewModel (MVVM), Single File Components (SFCs), and Composition API, outlining their benefits and demonstrating their application through practical examples. By understanding these best practices, you can elevate your Vue.js development skills and craft applications that are both elegant and efficient.
Introduction to Design Patterns
The Power of Patterns
Design patterns aren’t just for experienced architects and software engineers. In the context of software development, they represent reusable solutions to recurring problems. Think of them as blueprints or templates that capture proven solutions to common challenges. These patterns are shared knowledge, distilled from years of collective experience, allowing you to streamline your development process and build applications with greater confidence.
Why Design Patterns Matter in Vue.js
In a framework like Vue.js, where components and data flow are central to application logic, design patterns offer several key advantages:
- Improved Code Organization: Design patterns provide a structure for organizing your components and logic, making your code easier to understand, maintain, and extend.
- Enhanced Reusability: By adhering to established patterns, you can create components that are more easily reused across different parts of your application, reducing code duplication and improving efficiency.
- Enhanced Testability: Well-structured code based on design patterns is inherently easier to test, leading to more reliable and bug-free applications.
- Improved Collaboration: When developers on a team follow the same design patterns, it fosters a shared understanding of the codebase, making collaboration more effective and reducing conflicts.
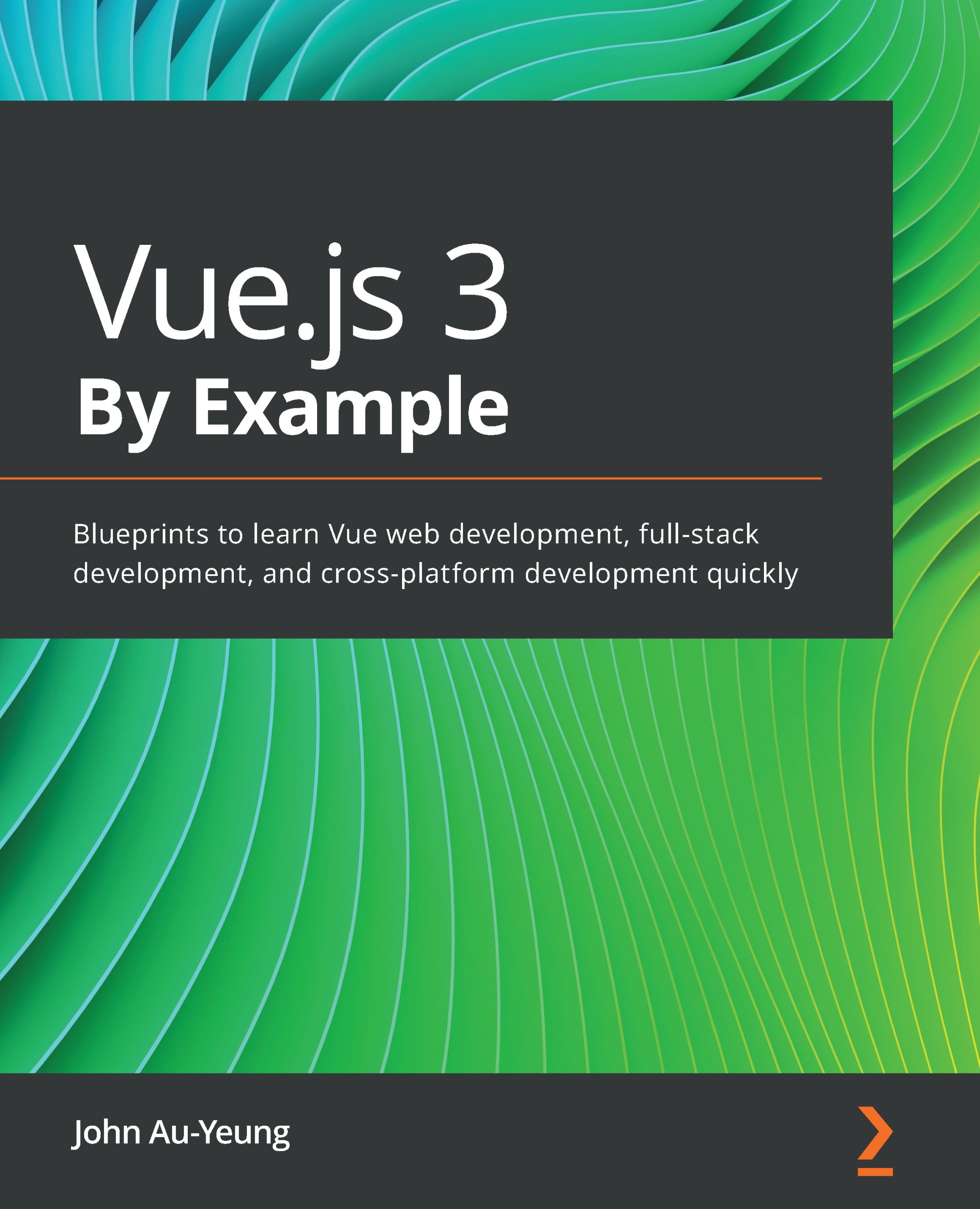
Image: www.packtpub.com
Common Design Patterns in Vue.js 3
1. Model-View-ViewModel (MVVM)
The MVVM Paradigm
MVVM, a popular architectural pattern, separates your application into three distinct parts:
- Model: Represents your data and business logic. Think of this as the core of your application’s functionality.
- View: The user interface, responsible for displaying information and handling user interactions. This is what your users see and interact with.
- ViewModel: A mediator that acts as a bridge between the Model and View. It exposes data and methods from the Model to the View, allowing the View to update itself based on changes in the data.
Benefits of MVVM
MVVM offers several advantages in Vue.js development:
- Separation of Concerns: Clearly separating data, logic, and presentation enhances code organization and maintainability, making it easier to debug and update your application.
- Improved Testability: Since each component is isolated and has defined responsibilities, testing becomes more straightforward, ensuring the quality of your application.
- Enhanced Data Binding: The ViewModel facilitates two-way data binding, automatically updating the View whenever changes occur in the data, and vice versa. This simplifies UI updates and results in a more responsive user experience.
Example: A Simple To-Do List
// Model: Todo.js
export default class Todo
constructor(title, completed = false)
this.title = title;
this.completed = completed;
// ViewModel (Vue Component): TodoList.vue
<template>
<ul>
<li v-for="(todo, index) in todos" :key="index">
<input type="checkbox" v-model="todo.completed">
<span :class=" completed: todo.completed "> todo.title </span>
</li>
</ul>
</template>
<script>
import Todo from './Todo';
export default
data()
return
todos: [
new Todo('Finish Vue.js project'),
new Todo('Write blog post'),
]
;
;
</script>
2. Single File Components (SFCs)
Organizing Your Code
SFCs are the cornerstone of Vue.js development, offering a convenient way to encapsulate a component’s entire logic within a single file. Each SFC typically contains:
- Template: The HTML structure of your component, defining the UI elements and how data is displayed.
- Script: JavaScript logic that powers your component, containing data, methods, and lifecycle hooks.
- Style: CSS styles that define the visual appearance of your component, ensuring styling stays localized within the component.
Advantages of SFCs
SFCs contribute significantly to a clean and organized project structure:
- Encapsulation: Each component becomes a self-contained unit, promoting code reuse and reducing dependencies between different parts of your application.
- Improved Maintainability: Changes to a component are confined to its SFC, minimizing the risk of unpredictable side effects in other parts of your application.
- Enhanced Development Workflow: SFCs streamline the development process by providing a single point of access for editing the template, logic, and styling of a component.
Example: A Simple Button Component
<template>
<button @click="handleClick"> text </button>
</template>
<script>
export default
props: ['text'],
methods:
handleClick()
// Logic to handle the button click
console.log('Button clicked!');
;
</script>
3. Composition API
Modernizing Vue Development
Vue.js 3 introduced the Composition API, offering a more flexible and modular way to structure your component logic. Unlike the Options API, which organizes code by properties like data, methods, and computed properties, the Composition API encourages grouping related logic into functions called ‘compositions’.
Benefits of the Composition API
The Composition API brings several advantages:
- Improved Logic Organization: Related logic (like fetching data, handling form submissions, or managing state) can be grouped within a single composition, enhancing readability and maintainability.
- Code Reuse: Compositions can be extracted into separate files and reused across multiple components, promoting code sharing and reducing redundancy.
- Enhanced Code Restructuring: The Composition API makes it simpler to move and rearrange code within a component, as it decouples logic from the component structure.
- Improved Testability: The modular nature of the Composition API simplifies unit testing, allowing you to test individual compositions in isolation.
Example: Using a Composition for Data Fetching
// fetch-data.js
import ref, onMounted from 'vue';
export default function useFetchData()
const data = ref(null);
const loading = ref(true);
const error = ref(null);
onMounted(async () =>
try
const response = await fetch('/api/data');
data.value = await response.json();
catch (err)
error.value = err;
finally
loading.value = false;
);
return
data,
loading,
error
;
// MyComponent.vue
<template>
<div v-if="loading">Loading...</div>
<div v-else-if="error">An error occurred.</div>
<div v-else> data </div>
</template>
<script>
import useFetchData from './fetch-data';
export default
setup()
const data, loading, error = useFetchData();
return data, loading, error ;
;
</script>
Vue.js 3 Best Practices
1. State Management: Pinia
State management becomes essential as your Vue.js applications grow in size and complexity. Pinia, an official Vue.js state management library, provides a streamlined and powerful way to manage application state. It offers features like mutable state, time-travel debugging, and easy data serialization, making it an excellent choice for state management in large-scale Vue.js projects.
2. Accessibility: Building Inclusive Applications
Accessibility is paramount. Ensure all users, regardless of abilities, can access and interact with your applications. Follow accessibility guidelines (WCAG, ARIA) when designing and developing your components. Use semantic HTML, ARIA attributes, and accessibility-focused libraries (like Vue.js Accessibility) to create truly inclusive user experiences.
3. Performance Optimization
Optimize your Vue.js applications for speed and efficiency. Use tools like the Vue.js Devtools to identify performance bottlenecks. Consider techniques like code splitting, lazy loading components, and using the `v-if` directive to conditionally render elements based on specific conditions. These optimizations can significantly improve the user experience, especially on mobile devices.
4. Testing for Reliability
Regularly test your Vue.js applications. Vue.js offers built-in support for unit testing (using frameworks like Jest) and end-to-end testing (using tools like Cypress). Write tests to verify component functionality, data management, and overall application behavior, ensuring the quality and robustness of your codebase.
5. Code Style & Linting
Maintain consistent code style and formatting. Use a linter (like ESLint) and a code formatter (like Prettier) to enforce style rules and prevent common errors that can lead to difficult-to-read and unpredictable code. This contributes to a more maintainable codebase and allows developers to focus on logic rather than minor formatting issues.
Vue.Js 3 Design Patterns And Best Practices Pdf
Conclusion: Building Future-Proof Vue.js Applications
By embracing design patterns and best practices, you can build Vue.js applications that are robust, scalable, and maintainable. Whether you’re a beginner or a seasoned developer, understanding these concepts will significantly elevate your Vue.js skillset. Remember, the patterns and practices discussed here are just a starting point. Continuously learn, experiment, and adapt your approach to future trends in the ever-evolving world of Vue.js. Invest in your development journey, and you’ll be well-equipped to create innovative and exceptional web experiences.